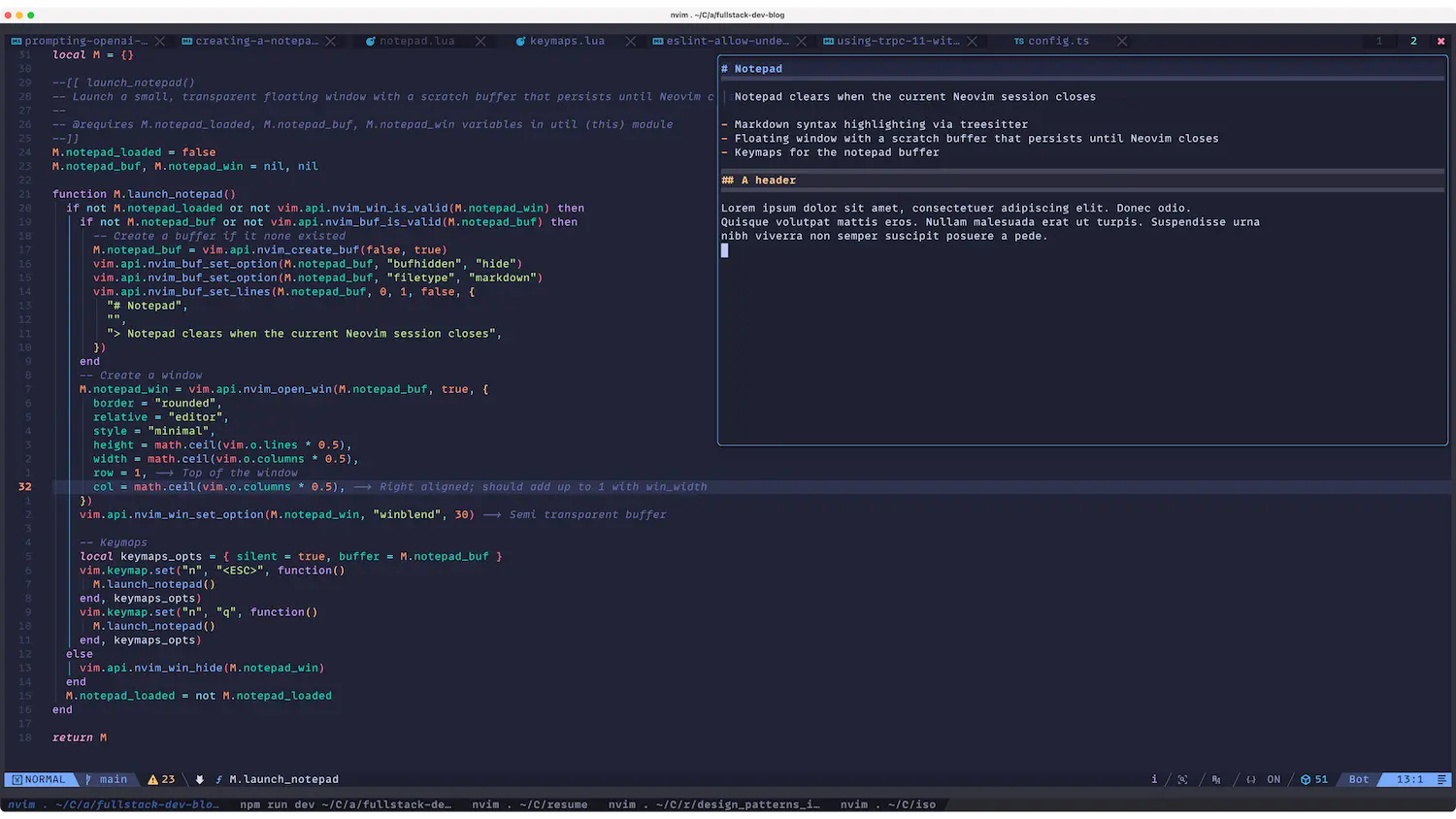
Creating a floating notepad in Neovim
Learn how to create a notepad in Neovim using the built-in window API.
:g :g/function /execute "norm cwconst^[f(i = f{i=> "
" continued...
execute "159,165norm 0fc2dw"
" continued...
const router = router({
create: publicProcedure
.input(z.object({ name: z.string() }))
.mutation(async (opts) => {
const { input } = opts;
const user = await prisma.user.create({
// continued...
const { data: user } = await trpc.user.create.mutate({ name: "Jane" });
// continued...
import prisma from "$services/prisma";
import type { FetchCreateContextFnOptions } from "@trpc/server/adapters/fetch";
interface CreateInnerContextOptions
extends Partial<FetchCreateContextFnOptions> {}
* @link https://trpc.io/docs/v11/context#inner-and-outer-context
*/
// continued...
import { initTRPC } from "@trpc/server";
import { createHTTPServer } from "@trpc/server/adapters/standalone";
import { appRouter } from "./router.ts";
import { createContext } from "./context.ts";
import cors from "cors";
createHTTPServer({
// continued...
interface MetricInput {
metricType: "views" | "gh_ratelimit_errors";
}
const router = router({
incrementMetric: publicProcedure.input(MetricInput).mutation((resolver) => {
const { prisma } = resolver.ctx;
// continued...
export const getRepoContents = async (props: {
owner: string;
repo: string;
path: string;
}) => {
const { owner, repo, path } = props;
// continued...
async (props: { owner: string; repo: string; path: string }) => {
const { owner, repo, path } = props;
try {
const body = await octokit.request(
"GET /repos/{owner}/{repo}/contents/{path}",
{
// continued...
import { initTRPC } from "@trpc/server";
import { z } from "zod";
import type { Context } from "./context";
import { getCommits, getRepoContents } from "./services/github";
export const t = initTRPC.context<Context>().create();
const { createCallerFactory, router } = t;
// continued...
import trpc from "$services/trpc";
import { createCaller } from "$rpc/router";
import prisma from "$services/prisma";
import type { APIRoute } from "astro";
export const GET: APIRoute = async ({ params, request, cookies }) => {
const queryParams = new URLSearchParams(request.url.split("?")[1]);
// continued...
vi.mock("../rpc-server/services/github.ts", () => {
return {
default: {
getRepoContents: (input: string) =>
new Promise((res) =>
res({
// continued...
describe("trpc router", () => {
it("should call the Github API", async () => {
const ctx = await createContext({
prisma: prismaMock,
githubService,
});
// continued...
const [_isZoomVisible, setZoomVisible] = useState(false);
// continued...
class BaseTransaction < ApplicationRecord
MIN_AMOUNT = 100
def min_amount
raise("Not implemented")
end
end
# continued...
class JobStatus < T::Enum
enums do
Pending = new
Running = new
Finished = new
end
# continued...
class RetryableJobStatusImpl < T::Enum
enums do
RetriesExhaused = new
end
end
RetryableJobStatus = T.type_alias { T.any(JobStatus, RetryableJobStatusImpl) }
# continued...
class RetryableJobHandler < Handler
extend T::Sig
extend T::Helpers
sig { override.returns(RetryableJobStatus) }
def run
if retries_exhausted?
# continued...
class JobStatus < T::Enum
enums do
Pending = new
Running = new
Finished = new
RetriesExhaused = new
# continued...
module Monetizable
extend ActiveSupport::Concern
class_methods do
def monetize(*fields)
fields.each do |field|
define_method("#{field}_money") do
# continued...
module Money::Arithmetic
CoercedNumeric = Struct.new(:value)
def +(other)
if other.is_a?(Money)
self.class.new(amount + other.amount, currency)
else
# continued...
class Money::Currency
include Comparable
class UnknownCurrencyError < ArgumentError
end
CONFIG = Rails.root.join("config/currencies.yml")
@@instances = {}
# continued...
irb(main):002> cur = Money::Currency.new("usd")
irb(main):003> cur.class.class_variable_get(:@@instances)
@default_format="%u%n",
# continued...
class Transaction < ApplicationRecord
include Monetizable
monetize :amount
end
# continued...
irb(main):005> t = Transaction.new(amount: 10, currency: 'usd')
=> #<Transaction:0x000000010f813c00 id: nil, amount: 0.1e2, currency: "usd", created_at: nil, updated_at: nil>
irb(main):014> t.amount_money
@amount=0.1e2,
@currency=
@default_format="%u%n",
# continued...
irb(main):006> t.amount_money + 10
=>
@amount=0.2e2,
@currency=
@default_format="%u%n",
@delimiter=",",
# continued...
irb(main):008> t.amount_money + Transaction.new(amount: 20, currency: 'usd').amount_money
=>
@amount=0.3e2,
@currency=
@default_format="%u%n",
@delimiter=",",
# continued...
class ExchangeRate < ApplicationRecord
include Provided
validates :base_currency, :converted_currency, presence: true
class << self
def find_rate(from:, to:, date:)
find_by \
# continued...
module ExchangeRate::Provided
extend ActiveSupport::Concern
class_methods do
private
def fetch_rate_from_provider(from:, to:, date:)
response = exchange_rates_provider.fetch_exchange_rate \
# continued...
def calculate(num)
return num * 2
end
# continued...
local M = {}
M.notepad_loaded = false
M.notepad_buf, M.notepad_win = nil, nil
M.notepad_buf = vim.api.nvim_create_buf(false, true)
vim.api.nvim_buf_set_option(M.notepad_buf, "bufhidden", "hide")
vim.api.nvim_buf_set_option(M.notepad_buf, "filetype", "markdown")
-- continued...
M.notepad_win = vim.api.nvim_open_win(M.notepad_buf, true, {
border = "rounded",
relative = "editor",
style = "minimal",
height = math.ceil(vim.o.lines * 0.5),
width = math.ceil(vim.o.columns * 0.5),
-- continued...
local keymaps_opts = { silent = true, buffer = M.notepad_buf }
vim.keymap.set("n", "<ESC>", function()
M.launch_notepad()
end, keymaps_opts)
vim.keymap.set("n", "q", function()
M.launch_notepad()
-- continued...
local M = {}
M.notepad_loaded = false
M.notepad_buf, M.notepad_win = nil, nil
function M.launch_notepad()
if not M.notepad_loaded or not vim.api.nvim_win_is_valid(M.notepad_win) then
if not M.notepad_buf or not vim.api.nvim_buf_is_valid(M.notepad_buf) then
-- continued...
map("n", "<leader>Ln", function()
require("util.notepad").launch_notepad()
end, { desc = "Toggle Notepad" })
-- continued...
* config.a = 1,
config.b = 2,
config.c = 3
-- continued...
{a = 1,b = 2,c = 3}
-- continued...
return {
{
"neovim/nvim-lspconfig",
opts = {
servers = {
ruby_ls = {},
-- continued...
vim.opt.sps = "file:/Users/aaron/.config/nvim/spell/sugg,best"
-- continued...
vim.cmd("set spell syntax=off")
-- continued...
{
"jackMort/ChatGPT.nvim",
dependencies = {
"MunifTanjim/nui.nvim",
"nvim-lua/plenary.nvim",
"nvim-telescope/telescope.nvim",
-- continued...
{
mods = "LEADER",
key = "1",
action = wezterm.action_callback(function(window, pane)
local home = wezterm.home_dir
local workspaces = {
-- continued...
{
key = "N",
mods = "CTRL|SHIFT",
action = a.PromptInputLine({
description = wezterm.format({
{ Attribute = { Intensity = "Bold" } },
-- continued...
{
"eslintConfig": {
"rules": {
"no-unused-vars": "off",
"@typescript-eslint/no-unused-vars": [
"warn",
/* continued...*/
{
"WriteRailsMethod": {
"type": "chat",
"opts": {
"template": "You are a Ruby on Rails programmer. Implement the {{method}} method(s) for the following code.\n\nExisting code:\n```{{filetype}}\n{{input}}\n```\n\n```{{filetype}}",
"title": "Write Rails code",
/* continued...*/
{
body: {
status: 200,
url: 'https://api.github.com/repos/facebook/react/contents/packages%2Fshared%2FshallowEqual.js',
headers: {
'x-ratelimit-limit': '60',
/* continued...*/
"eslint: '\_myVar' is assigned a value but never used"
# continued...
StylesPath = .vale/styles
MinAlertLevel = suggestion
Packages = write-good, proselint, Microsoft # `vale sync` will download these packages for you.
Vocab = Me
[*]
IgnoredScopes = code, tt, table, tr, td # ignore HTML
# continued...
For the following instructions, do not use \"get :create\" etc and
instead use full routes like \"api_v1_birds_path\". Implement RSpec tests for
the following code.\n\nCode:\n```{{filetype}}\n{{input}}\n```\n\nTests:\n```{{filetype}}
// continued...
You are a Ruby on Rails programmer.
Implement the {{method}} method(s) for the following code.\n\nExisting
code:\n```{{filetype}}\n{{input}}\n```\n\n```{{filetype}}
// continued...
usd:
name: United States Dollar
priority: 1
iso_code: USD
iso_numeric: "840"
html_code: "$"
# continued...
npm install @trpc/server@next @trpc/client@next @trpc/react-query@next @trpc/next@next @tanstack/react-query@latest @tanstack/react-query-devtools@latest
# continued...